SOLID Principles in PHP : Learn how to write better code
- Description
- Curriculum
- FAQ
- Reviews
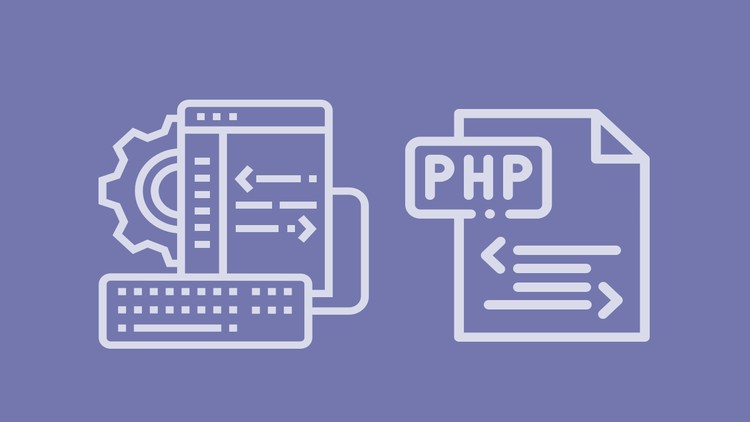
Object Oriented Design principles, SOLID, either you love them or hate them. However, they are the crucial building blocks of your software’s architecture. Unfortunately, as useful as they are, most of the material available on this subject is either too abstract or use impractical examples. Now, they do help in understanding these principles conceptually, but they lack the depth and understanding as how and where these principles can benefit you the most.
Well, in this course, I will walk you through these principles step by step. I have structured the course in such a way that by the end of it, not only you will have completely understood these mystical concepts but also you will be ready to apply them in your projects straight away. I will also show you how these principles can rescue you from the darkness of messy code by giving you practical examples.
In addition to that, I will also show you a few tips and tricks along the way in Laravel and a gentle introduction to testing.
-
3Introducing Single Responsibility Principle
In this lesson, I will introduce the first letter S in SOLID. Then we will look at a very general example to have a better conceptual understanding of the principle.
-
4Walk-through of a real world use case
In this lesson, We will look at a contrived example imitating a very much real world use case. We will write a test and make sure that test is passing before we start applying SRP
-
5Lets apply Single Responsibility Principle
In this lesson, we will start refactoring our code from previous example utilizing Single Responsibility Principle. We will make sure that our tests are returning green while we are refactoring
-
6Test your SRP Knowledge
-
7Introducing Open Closed Principle
In this lesson, I will introduce the letter O in SOLID. We will have a detailed discussion about this principle as how and why its an important principle
-
8Walk-through of a contrived example
In this lesson, I am going to walk you through a very contrived example AreaCalculator, which will help us understand OCP conceptually
-
9Real world use case of using OCP - Part 1
In this lesson, I will walk you through a real world example where we have received business requirements and we need to refactor the code. Part 1 of 2 parts lesson
-
10Real world use case of using OCP - Part 2
This is part 2 of 2 parts lesson, where we will conform to OCP
-
11Test your OCP Knowledge
-
12Introducing Liskov Substitution Principle
In this lesson I will introduce you to Liskov Substitution Principle and what does it state
-
13Breaking down LSP , using real world use case - Part 1
In this lesson we will break down LSP's rules by walking through a real world use case.
-
14Breaking down LSP's technical jargon
In this lesson we will look at the strict definition of LSP. We will then talk about three rules that one must meet to fully conform to LSP. By the end of this lesson, you will have a fairly good understanding of this principle.
-
15Test your newly acquired LSP knowledge
-
16Introducing Interface Segregation Principle
In this lesson I will introduce you to Interface Segregation Principle and walk you through a very contrived example to understand the concept better
-
17Using Interface Segregation Principle in real world -Part 1
This is part 1 of 2 parts lesson. In this lesson, i will walk you through a fictitious Online Book Store and in particular its order manger .
-
18Using Interface Segregation Principle in real world -Part 2
This is part 2 of 2 parts lesson. In this lesson we will carry on with our Order Manager and finally make it conform to ISP.
-
19Test your newly acquired ISP knowledge
-
20Introducing Dependency Inversion Principle - DIP
In this lesson, I will introduce you to Dependency Inversion Principle. I will also reveal that we have been secretly utlizing this principle in our previous examples
-
21Using Dependency Inversion Principle in real world
In this lesson, I will take you back to OrderProcessing Service from first lesson and we will use DIP to solve the payment service
-
22Test your newly acquired Dependency Inversion Principle knowledge