Code Your First Game: Arcade Classic in JavaScript on Canvas
- Description
- Curriculum
- FAQ
- Reviews
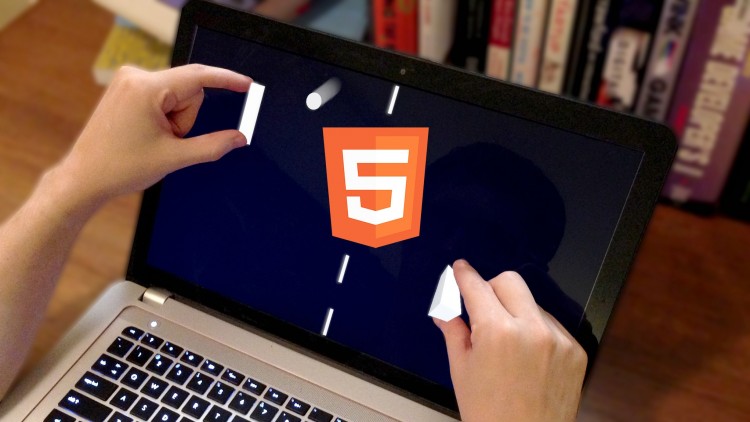
At the end of this short course you’ll have programmed your first game. You’ll learn gameplay development fundamentals by really doing it – writing and running real code on your own machine.
Each step of the course has the source code attached exactly as it should look at that time (click “View Resources” then “Downloadable Resources”), for you to compare to or pick up from, so you can’t get stuck!
Begin Your Game Programming Journey the Proven Way
“Make the simplest game possible.” “Program a ball and paddle project.” “Practice by first remaking something from the 1970’s.” All beginning developers hear this advice from more experienced peers… because it works!
By following this approach you will:
- Learn design from a fun classic that people know and enjoy.
- Start your practice today – now! – without waiting for an idea.
- Finish your game in hours or in a weekend, not over months.
- Understand every line of code used in the entire program.
- Avoid distraction from searching for or creating detailed art.
- Master fundamentals needed to make your own games better.
You can program this game with a normal text editor, and run it in the web browser you already have. No special software is needed.
Though you’ll be coding in JavaScript for HTML5 canvas in this course, the focus is on common game programming concepts. You can later apply these same patterns to get quick results in other programming languages such as C#, Java, ActionScript 3, C++, or Python.
I’m a private game development trainer, and for clients new to gameplay programming this is exactly the material that I cover to get them started quickly. Within hours you will have finished programming your first project. This is the fastest way to get results. The momentum gained from doing this provides a solid foundation to give more advanced concepts meaning and context as you continue on in your journey of learning game development.
(HTML5 Logo in the course image is by W3C, licensed under Creative Commons Attribution 3.0 Unported.)
-
1Introduction and First Code File
By following along with this video you'll create your first code file by using a plain text editor, and you'll test run that code by using any common web browser. You'll also see how to view your browser's console, which will be very useful for troubleshooting errors later.
In case you get stuck at any point in this video – or any one later in this course – you can download each video's example source code zip file in order to have a snapshot of exactly how the code ought to look after completing each step covered in this course.
-
2Drawing and Positions
You'll learn how to draw, position, and change the dimensions of rectangles on the screen. This drawing technique will soon be used to represent both paddle positions.
-
3Movement and Time
Live action with motion requires that the code doesn't just happen once – it has to keep running over and over again. In this step you'll get that working, and by doing so you'll open up a whole world of possibilities for dynamic movements and interactivity.
-
4Clean Up the Code
For this brief intermission we'll take a few moments to rethink how the functionality has been programmed so far. These changes will not affect what the game does, but they will make the upcoming changes much easier. This type of "refactoring" as the program grows is a common part of programming.
-
5Bouncing the Ball
Here you'll write code to detect when the ball is crossing an edge of the graphics canvas. As it does so, you'll reverse its direction to bounce it off the boundaries, keeping the ball in play and in view.
-
6Circle Draw Details
Drawing a circle is a bit more involved than drawing a rectangle, but the game will look much better with a round ball. You'll learn in this step how to display a filled circle on the screen. Next you'll hide all the complex details of doing so in a new helper function, which will greatly reduce how much you'll need to remember or figure out for the next time that you need to draw a circle.
-
7Ball 2D Motion, Paddle
So far, the ball only moved side-to-side. Now with this step you'll move and bounce the ball vertically, as well. You'll also hook up the paddle's position to follow your mouse input.
-
8Ball Reset and Collision
You'll now write the ball collision code for each paddle, causing the ball to reset instantly whenever it collides with the left or right side unless it's striking an area blocked by the paddle.
-
9Paddle AI and Scoring
Here you'll program the right paddle to move and play automatically by implementing a basic form of artificial intelligence. You'll also add scoring to the game, giving your player a way to see how well he or she is performing against the computer opponent.