Learn PHP Programming From Scratch
- Description
- Curriculum
- FAQ
- Reviews
- Grade
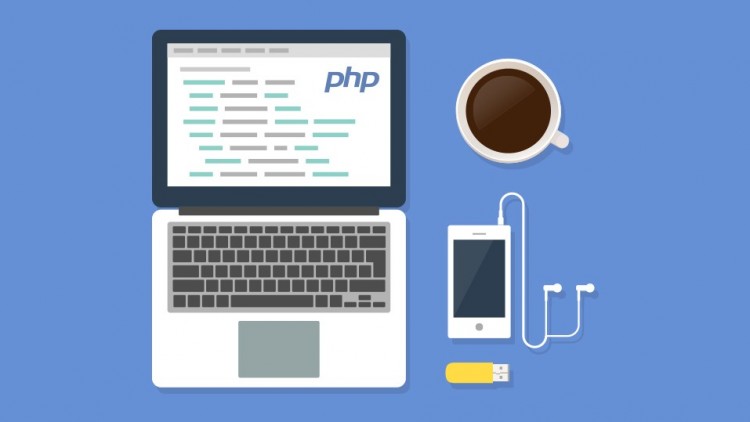
Do you want to be a web developer? Do you need to brush up on your PHP skills? Then you’re in the right place!
This is the most comprehensive PHP course on Udemy. You will learn everything from the basics to more advanced PHP programming using real world examples and sample projects.
Check out the free sample videos to get a free taste of PHP!
This course is updated regularly with new content and new projects to work on, so even if you get through the entire course, there will always be more the next time you log in.
What Is PHP?
PHP is an open source (free) scripting language that allows you to create dynamic websites and work with servers. PHP is now used on over 20 million websites and over 1 million servers worldwide.
-
4Commenting Code
-
5Setting Up A Web Server
-
6Echo/Print
The echo language construct allows you to output data to the browser. Also, a quick word about the print language construct.
* Section 2 source codes can be downloaded from the right side of the page.
-
7Variables
How to store values into variables, the rules for variable naming and how to output them to the user
-
8If StatementsA look at IF, IF ELSE and IF ELSEIF ELSE statements, with a example to help you understand the uses. Uses comparison operators in the example, which you can learn more about from our other videos.
-
9Arithmetic OperatorsThe arithmetic operators within PHP, with examples using them. Talks about addition, subtraction, multiplication, division, modulus (remainder), increment and decrement.
-
10Comparison OperatorsComparison operators allow you to compare values. For example, is the person old enough to do something?
-
11Triple EqualsThe triple equal (===) compares not only values, but data types too.
-
12Logical OperatorsA look at logical operators, and detailed examples of how and why we use them. The use of NOT is outlined in my other videos.
-
13Switch StatementThe switch statement offers a faster, cleaner way of comparison. This tutorial provides a couple of examples of using the switch statement.
-
14ArraysHow to create an array, and assign values to either default or defined keys. Also speaks about foreach construct briefly.
-
15Multi-dimensional ArraysCreating a multi-dimensional array following on from the previous example in the array video tutorial by phpacademy. This will show you how to create up to 3 dimensions, with an example of being able to store properties for each name
-
16While LoopThe while loop, it's syntax, and an example of counting numbers.
-
17Do While LoopThe do while loop, it's syntax, how it differs from a while loop, and an example of counting numbers
-
18For LoopsThe for loop, it's syntax, and an example of counting numbers.
-
19ForeachExplains the use of foreach construct for obtaining keys and values from an array, and displaying them
-
20FunctionsWhy functions are useful, how to declare them, and a few examples.
-
21Functions with Undefined ParametersFunction parameters don't need to be defined, and the use of this method means you can pass as many values to a function without defining them
-
22Formatting NumbersFormat decimal points, or display numbers in thousands, etc. with commas to separate. We also look at reducing the amount of characters after the decimal point of a number.
-
23$_GET$_GET allows values to be read in through the page URL (e.g. index.php?name=Alex). This example shows how to read these in, and how to incorporate a form as to submit these variables
-
24$_POST$_POST allows values to be read in through the HTTP POST method. This example shows how to read these in, and how to incorporate a form as to submit these variables.
-
25Embedding PHP within HTMLHow to embed PHP inside HTML, including an example with a form and a textarea. Please ensure you use the htmlentities() function, wrapped around your $_POST['name'], when submitting data to be displayed on a page
-
26A better way to display HTMLA much better, faster way of displaying HTML within PHP. In this example, in an IF ELSE statement.
-
27Arrays (Part 1/2)A more in depth look at arrays with some examples. Includes, creating and adding to arrays, dumping array contents and creating a function to output values from a specific array
-
28Arrays (Part 2/2)A more in depth look at arrays with some examples. Includes, creating and adding to arrays, dumping array contents and creating a function to output values from a specific array.
-
29Cookies (Part 1)Cookies store data on the users computer ready for the server to read back from them. I show you how to set, show, check for and destroy cookies in this tutorial
-
30Cookies (Part 2)Cookies store data on the users computer ready for the server to read back from them. I show you how to set, show, check for and destroy cookies in this tutorial
-
31CheckboxesAn introduction to submitting and processing checkboxes with PHP.
-
32Radio ButtonsAn introduction to submitting and processing radio buttons with PHP.
-
33Sessions
Simelar to cookies, sessions store data, but data is destroyed when the connection is lost (i.e. browser is closed). I show you how to create, show, and unset sessions.
-
34Explode (String to Array)Exploding a string will split a string by a specific character (a delimiter) and place each broken string into an element within an array. This tutorial covers the basic concept of exploding strings with PHP, with an example.
-
35Implode (Array to String)Imploding an array will take each element, and create a string, including a specified delimiter. This tutorial covers the basic concept of imploding arrays with PHP, with an example
-
36Ternary Operator (Inline IF statement)The ternary operator is essentially an inline IF statement, saving a lot of time and lines of code. In this tutorial we shorten around 9 lines, to 2.
-
37Quickly Return True or FalseQuickly return true or false from a function. A handy tip that should save some time!
-
38Splitting A String By Spaces (Whitespace)Splitting a string by an unlimited (or, large) amount of spaces. Useful for splitting up search terms to process keywords. We use a very simple regular expression here, in conjunction with the preg_split function
-
39Generate a Random NumberSimply generate a random number with PHP.
-
40Introduction Connecting to a database with PDO, using one of the available PDO drivers. In this case, we're connecting using MySQL.
-
41Listing available driversNow we've connected to MySQL and selected our database using PDO, we need to query our database and return a result set. We look at returning all results, or results that can be looped through (fetching next row with each loop). We also look at returning in both associative and numerical arrays.
-
42Setting up databaseUsing a PDOStatement method, we can return the amount of rows that were affected by our last query, which can be very useful!
-
43ConnectingBinding values to a query offers SQL injection protection. Here, we look at an example of searching a database table for user defined input, and injecting this value into the query.
-
44Error levelsWe can set the error reporting attribute of the PDO object so methods throw exceptions on error. We can then make use of a try catch block to catch thrown exceptions.
-
45Basic queryHere, we look at simply returning the last inserted ID for the last executed query
-
46Fetching resultsTransactions offer the benefit of 'storing' a sequence of queries to be committed. This benefit means we can roll back these queries if an exception is thrown.
-
47Looping
-
48Fetch all
-
49Fetch as object
-
50Row count
-
51Project - user class dates
-
52Escaping
-
53Prepared statements
-
54Inserting
-
55Last inserted id
-
56Introduction
-
57Conecting to a database
-
58Connecting using php
-
59Creating database table
-
60Pulling single record
-
61Pulling multiple records
-
62Different result types
-
63Counting results
-
64Updating and affected rows
-
65Escaping
-
66Prepared statements for inserting
-
67Selecting prepared statements
-
68Free result
-
69Closing db connection
-
70Introduction to Our Project
-
71Installing Laravel
-
72The Database
-
73Creating the Home Page
-
74Templating
-
75PDO and SMTP E-mail
-
76Creating Accounts Part 1
-
77Creating Accounts Part 2
-
78E-mail Activation
-
79Signing In
-
80Signing Out
-
81Remember Me
-
82Changing Passwords
-
83User Profiles
-
84Account Recovery
-
85Migrations